CLEAR ENTRY
This function will allow you to scan through a provided array for a provided entry, and if it finds such a entry, will remove it.
There is a built in remove(index)
ability you can also use to remove array entries too, which this function actually uses.
However for that you need to provide the index number, while there may be instances where you want to remove an entry by it's actual data instead.
To install.
copy & paste the following code into the Global Functions panel of Game Configuration in your project.
function clear_entry($array, $entry) begin
$index = 0;
for $i in $array do
if $i == $entry then
$array.remove($index);
break;
end;
$index += 1;
end;
end;
To use,
simply call the function and provide the array to search, and the data to search for.
Example:
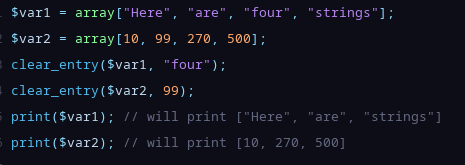
By default, this function will find and delete the first entry it finds that matches the provided data, and then stop.
If you wish to find and remove all entries that match the provided data, simply replace break;
from the function code with $index -= 1;
.
The result would be something like this:
